hai friends in this tutorial we will implement simple chat application using firebase in very simple way
features of this application :
- Login functionality
- Register functionality
- Chatting functionality
- Group chat functionality
final output :
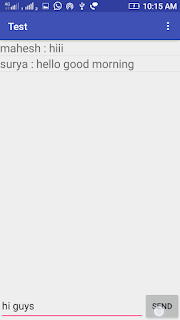 |
chat page |
Lets start our work
- create a new android project in android studio
- setup the firebase with our android project (if you don't know about please see the below video)
- add the following dependencies in our project under project structure build.gradle(Module: app) file in module section
compile 'com.google.firebase:firebase-database:10.0.0'
compile 'com.google.firebase:firebase-auth:10.0.0'
compile 'com.firebaseui:firebase-ui-database:0.6.2'
create User Interface for our Application
- make our activity_main.xml file like below (under res/layout/activity_main.xml)
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:orientation="vertical"
android:layout_height="match_parent"
>
<ListView
android:layout_width="match_parent"
android:layout_height="0dp"
android:id="@+id/listview"
android:layout_weight="0.9">
</ListView>
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_weight="0.1"
android:layout_height="0dp"
>
<EditText
android:layout_width="0dp"
android:layout_height="match_parent"
android:id="@+id/edittext"
android:layout_weight="0.8"/>
<Button
android:layout_width="0dp"
android:layout_height="match_parent"
android:text="Send"
android:onClick="send"
android:layout_weight="0.2"
/>
</LinearLayout>
</LinearLayout>
Creating LoginActivity
- right click on the package (example: com.example.mahesh.test)
- then New->Activity->Empty Activity
- give name as "LoginActivity"
make our activity_login.xml file like below (under res/layout/activity_login.xml)
activity_login.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/activity_login"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.example.mahesh.test.LoginActivity">
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textPassword"
android:ems="10"
android:layout_marginTop="34dp"
android:id="@+id/password"
android:hint="password.."
android:layout_below="@+id/email"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
/>
<Button
android:text="Login"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:onClick="login"
android:layout_marginTop="69dp"
android:id="@+id/login"
android:layout_below="@+id/password"
android:layout_centerHorizontal="true" />
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textPersonName"
android:hint="email.."
android:ems="10"
android:layout_marginTop="109dp"
android:id="@+id/email"
android:layout_alignParentTop="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
/>
<TextView
android:text="new user?"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:onClick="register"
android:id="@+id/newUser"
android:layout_below="@+id/button2"
android:layout_alignRight="@+id/button2"
android:layout_alignEnd="@+id/button2"
android:layout_marginTop="37dp" />
</RelativeLayout>
Creating RegisterActivity
- right click on the package (example: com.example.mahesh.test)
- then New->Activity->Empty Activity
- give name as "RegisterActivity"
make our activity_register.xml file like below (under res/layout/activity_register.xml)
activity_register.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/activity_register"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.example.mahesh.test.RegisterActivity">
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textPersonName"
android:hint="email"
android:ems="10"
android:layout_marginTop="62dp"
android:id="@+id/email"
android:layout_alignParentTop="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true" />
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textPersonName"
android:hint="name"
android:ems="10"
android:layout_below="@+id/email"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:layout_marginTop="24dp"
android:id="@+id/name" />
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textPassword"
android:hint="password"
android:ems="10"
android:layout_marginTop="22dp"
android:id="@+id/password"
android:layout_below="@+id/name"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true" />
<Button
android:text="Submit"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:onClick="submit"
android:layout_centerHorizontal="true"
android:layout_marginBottom="93dp"
android:id="@+id/button3" />
</RelativeLayout>
creating individual_row.xml
- right click on layout
- new->Layout resource file
- give name as "individual_row.xml"
make our individual_row.xml file like below (under res/layout/individual_row.xml)
individual_row.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical" android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:text="TextView"
android:layout_width="match_parent"
android:textSize="20sp"
android:layout_height="wrap_content"
android:id="@+id/textView1" />
</LinearLayout>
MainActivity.java
package com.example.mahesh.test;
import android.os.*;
import android.support.v7.app.AppCompatActivity;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.EditText;
import android.widget.ListView;
import android.widget.TextView;
import com.firebase.ui.database.FirebaseListAdapter;
import com.google.firebase.auth.FirebaseAuth;
import com.google.firebase.database.DataSnapshot;
import com.google.firebase.database.DatabaseError;
import com.google.firebase.database.DatabaseReference;
import com.google.firebase.database.FirebaseDatabase;
import com.google.firebase.database.ValueEventListener;
import java.util.HashMap;
public class MainActivity extends AppCompatActivity {
private EditText editText;
private DatabaseReference chat_data_ref;
private DatabaseReference user_name_ref;
private ListView listView;
private FirebaseAuth mAuth;
private String name="";
HashMap<String,String> map;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mAuth= FirebaseAuth.getInstance();
editText=(EditText)findViewById(R.id.edittext);
chat_data_ref= FirebaseDatabase.getInstance().getReference().child("chat_data");
user_name_ref=FirebaseDatabase.getInstance().getReference().child("chat_users").child(mAuth.getCurrentUser().getUid()).child("name");
listView=(ListView)findViewById(R.id.listview);
map=new HashMap<>();
user_name_ref.addValueEventListener(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
// This method is called once with the initial value and again
// whenever data at this location is updated.
name=dataSnapshot.getValue().toString();
}
@Override
public void onCancelled(DatabaseError error) {
// Failed to read value
}
});
FirebaseListAdapter<Message> adapter=new FirebaseListAdapter<Message>(
this,Message.class,R.layout.individual_row,chat_data_ref
) {
@Override
protected void populateView(View v, Message model, int position) {
TextView msg=(TextView)v.findViewById(R.id.textView1);
msg.setText(model.getUser_name()+" : "+model.getMessage());
}
};
listView.setAdapter(adapter);
}
public void send(View view) {
chat_data_ref.push().setValue(new Message(editText.getText().toString(),name));//storing actual msg with name of the user
editText.setText("");//clear the msg in edittext
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.menu,menu);
return super.onCreateOptionsMenu(menu);
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
if(item.getItemId()==R.id.logout)
{
mAuth.signOut();//when the user clicks signout option this will executes
finish();
}
return super.onOptionsItemSelected(item);
}
}
LoginActivity.java
package com.example.mahesh.test;
import android.app.ProgressDialog;
import android.content.Intent;
import android.support.annotation.NonNull;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.EditText;
import android.widget.Toast;
import com.google.android.gms.tasks.OnCompleteListener;
import com.google.android.gms.tasks.Task;
import com.google.firebase.auth.AuthResult;
import com.google.firebase.auth.FirebaseAuth;
import com.google.firebase.auth.FirebaseUser;
public class LoginActivity extends AppCompatActivity {
private static final String TAG = "LoginActivity";
private EditText email,password;
private FirebaseAuth mAuth;
private FirebaseAuth.AuthStateListener mAuthListener;
private ProgressDialog loginDialog;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
email=(EditText)findViewById(R.id.email);
password=(EditText)findViewById(R.id.password);
mAuth=FirebaseAuth.getInstance();
loginDialog =new ProgressDialog(this);
loginDialog.setMessage("Login..");
mAuthListener = new FirebaseAuth.AuthStateListener() {
@Override
public void onAuthStateChanged(@NonNull FirebaseAuth firebaseAuth) {
if (firebaseAuth.getCurrentUser() != null) {
startActivity(new Intent(LoginActivity.this, MainActivity.class).addFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP | Intent.FLAG_ACTIVITY_NEW_TASK));
finish();
}
}
};
}
@Override
public void onStart() {
super.onStart();
mAuth.addAuthStateListener(mAuthListener);
}
public void login(View view) {
loginDialog.show();
mAuth.signInWithEmailAndPassword(email.getText().toString(),password.getText().toString()).addOnCompleteListener(new OnCompleteListener<AuthResult>() {
@Override
public void onComplete(@NonNull Task<AuthResult> task) {
if (!task.isSuccessful()) {
loginDialog.dismiss();
Toast.makeText(getApplicationContext(), "Authentication failed.",
Toast.LENGTH_SHORT).show();
}
loginDialog.dismiss();
}
});
}
public void register(View view) {
startActivity(new Intent(LoginActivity.this,RegisterActivity.class));
finish();
}
}
RegisterActivity.java
package com.example.mahesh.test;
import android.app.ProgressDialog;
import android.support.annotation.NonNull;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
import android.widget.Toast;
import com.google.android.gms.tasks.OnCompleteListener;
import com.google.android.gms.tasks.OnSuccessListener;
import com.google.android.gms.tasks.Task;
import com.google.firebase.auth.AuthResult;
import com.google.firebase.auth.FirebaseAuth;
import com.google.firebase.database.DatabaseReference;
import com.google.firebase.database.FirebaseDatabase;
public class RegisterActivity extends AppCompatActivity {
private EditText email,name,password;
DatabaseReference databaseReference;
FirebaseAuth mAuth;
private DatabaseReference userIdRef;
ProgressDialog registerDialog;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_register);
email=(EditText)findViewById(R.id.email);
name=(EditText)findViewById(R.id.name);
password=(EditText)findViewById(R.id.password);
databaseReference= FirebaseDatabase.getInstance().getReference().child("chat_users");
mAuth=FirebaseAuth.getInstance();
registerDialog=new ProgressDialog(this);
registerDialog.setMessage("Registering..");
}
public void submit(View view) {
registerDialog.show();
mAuth.createUserWithEmailAndPassword(email.getText().toString(),password.getText().toString()).addOnCompleteListener(this, new OnCompleteListener<AuthResult>() {
@Override
public void onComplete(@NonNull Task<AuthResult> task) {
if(task.isComplete())
{
registerDialog.dismiss();
Toast.makeText(getApplicationContext(),"Registered successfully",Toast.LENGTH_SHORT).show();
userIdRef=databaseReference.child(mAuth.getCurrentUser().getUid());
userIdRef.child("name").setValue(name.getText().toString());
finish();
}
registerDialog.dismiss();
}
});
}
}
Message.java
21 comments
The 'Message' class will be extends from which class?
Message class is not extend from any class that is only create for model(getting the data from firebase)
hi! can u help me? when i download the apk, the moment i register the app crash
Only database is created in firebase with user info. The message activity isn't opening. It gets crashing all the time
Where do I put the Message.java ?
At the time of registering the app is crashing .what should i do overcome it ?
where is the project zip?
and/or apk
Thank you for sharing this article, it is very easy to understand and informative. Excellent!
Good tutorial and good programing codes you have used.it's useful.
Best Mobile Applications Company in Hyderabad
dear if we want to share files than what will we do
At the time of registering the app is crashing .what should i do overcome it ? and The message activity isn't opening. It gets crashing all the time
get username error is not resolve in main activity what i do please help
thanks for sharing this information
Blue Prism Training in Bangalore
Blue Prism Training in BTM
informatica Training in BTM
informatica Training in Bangalore
MEAN Stack Training in BTM
MEAN Stack Training in Bangalore
msg send are not displaying on emulator screen
yurtdışı kargo
resimli magnet
instagram takipçi satın al
yurtdışı kargo
sms onay
dijital kartvizit
dijital kartvizit
https://nobetci-eczane.org/
PBMD
Hollanda yurtdışı kargo
İrlanda yurtdışı kargo
İspanya yurtdışı kargo
İtalya yurtdışı kargo
Letonya yurtdışı kargo
CDK
Litvanya yurtdışı kargo
Lüksemburg yurtdışı kargo
Macaristan yurtdışı kargo
Malta yurtdışı kargo
Polonya yurtdışı kargo
JİNN
Portekiz yurtdışı kargo
Romanya yurtdışı kargo
Slovakya yurtdışı kargo
Slovenya yurtdışı kargo
İngiltere yurtdışı kargo
UQQEC
Yunanistan yurtdışı kargo
Afganistan yurtdışı kargo
Amerika Birleşik Devletleri yurtdışı kargo
Amerika Samoası yurtdışı kargo
Angola yurtdışı kargo
KFPJ
Emoticon Emoticon